When Are Enum Values Defined?
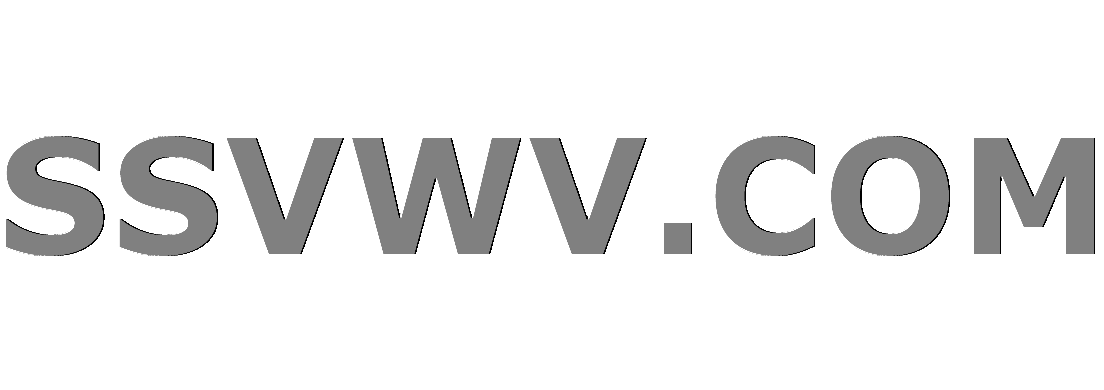
Multi tool use
I'm trying to implement something in a semi-efficient manner. In my program, I have an enumerator representing metrics that are used to pass information around in the program.
I have a class that lets me "compose" these metrics into a single object so I can essentially create a "sentence" to give me complex information without having to do any complex parsing of information. They're essentially bit flags but since I'm using an enum, I can have more than just 64 of them.
The problem is that if I'm instantiating instances of this container class a lot, and I go to create an array like so:
metrics = new boolean[Metrics.values().length];
I feel like creating an array of the enumerated values so often just to request its size is wasteful. I'm wondering if it's possible to just define the size of the enumerated values() in a static context so it's a constant value I don't have to recalculate:
private static final int METRIC_COUNT = Metrics.values().length;
Can I do this? Or would the compiler not have defined the enumerated values before it declares this static variable? I know this isn't the best explanation of my question, but I'm not sure how else to word it.
I know the static variable will be determined at runtime (unless the value assigned to it were a literal value), so would the program be able to request the members of the Metrics enum at this point in execution?
java
add a comment |
I'm trying to implement something in a semi-efficient manner. In my program, I have an enumerator representing metrics that are used to pass information around in the program.
I have a class that lets me "compose" these metrics into a single object so I can essentially create a "sentence" to give me complex information without having to do any complex parsing of information. They're essentially bit flags but since I'm using an enum, I can have more than just 64 of them.
The problem is that if I'm instantiating instances of this container class a lot, and I go to create an array like so:
metrics = new boolean[Metrics.values().length];
I feel like creating an array of the enumerated values so often just to request its size is wasteful. I'm wondering if it's possible to just define the size of the enumerated values() in a static context so it's a constant value I don't have to recalculate:
private static final int METRIC_COUNT = Metrics.values().length;
Can I do this? Or would the compiler not have defined the enumerated values before it declares this static variable? I know this isn't the best explanation of my question, but I'm not sure how else to word it.
I know the static variable will be determined at runtime (unless the value assigned to it were a literal value), so would the program be able to request the members of the Metrics enum at this point in execution?
java
2
You can certainly cache the length statically. Though I don't see where the array comes in. If you want to mimic a bit field, considerEnumSet
.
– shmosel
2 hours ago
2
stackoverflow.com/a/32354397/2970947
– Elliott Frisch
2 hours ago
1
Further to @ElliotFrisch's link, callingvalues()
does create a copy of the enum's array (as opposed to exposing the Enum's own private one, which would open it to being modified).
– racraman
1 hour ago
FYI, theenum
facility in Java (based onEnum
class) is usually just called “enum” or “enum type” to avoid confusion with the interfacejava.util.Enumeration
.
– Basil Bourque
1 hour ago
add a comment |
I'm trying to implement something in a semi-efficient manner. In my program, I have an enumerator representing metrics that are used to pass information around in the program.
I have a class that lets me "compose" these metrics into a single object so I can essentially create a "sentence" to give me complex information without having to do any complex parsing of information. They're essentially bit flags but since I'm using an enum, I can have more than just 64 of them.
The problem is that if I'm instantiating instances of this container class a lot, and I go to create an array like so:
metrics = new boolean[Metrics.values().length];
I feel like creating an array of the enumerated values so often just to request its size is wasteful. I'm wondering if it's possible to just define the size of the enumerated values() in a static context so it's a constant value I don't have to recalculate:
private static final int METRIC_COUNT = Metrics.values().length;
Can I do this? Or would the compiler not have defined the enumerated values before it declares this static variable? I know this isn't the best explanation of my question, but I'm not sure how else to word it.
I know the static variable will be determined at runtime (unless the value assigned to it were a literal value), so would the program be able to request the members of the Metrics enum at this point in execution?
java
I'm trying to implement something in a semi-efficient manner. In my program, I have an enumerator representing metrics that are used to pass information around in the program.
I have a class that lets me "compose" these metrics into a single object so I can essentially create a "sentence" to give me complex information without having to do any complex parsing of information. They're essentially bit flags but since I'm using an enum, I can have more than just 64 of them.
The problem is that if I'm instantiating instances of this container class a lot, and I go to create an array like so:
metrics = new boolean[Metrics.values().length];
I feel like creating an array of the enumerated values so often just to request its size is wasteful. I'm wondering if it's possible to just define the size of the enumerated values() in a static context so it's a constant value I don't have to recalculate:
private static final int METRIC_COUNT = Metrics.values().length;
Can I do this? Or would the compiler not have defined the enumerated values before it declares this static variable? I know this isn't the best explanation of my question, but I'm not sure how else to word it.
I know the static variable will be determined at runtime (unless the value assigned to it were a literal value), so would the program be able to request the members of the Metrics enum at this point in execution?
java
java
asked 2 hours ago
Darin BeaudreauDarin Beaudreau
191116
191116
2
You can certainly cache the length statically. Though I don't see where the array comes in. If you want to mimic a bit field, considerEnumSet
.
– shmosel
2 hours ago
2
stackoverflow.com/a/32354397/2970947
– Elliott Frisch
2 hours ago
1
Further to @ElliotFrisch's link, callingvalues()
does create a copy of the enum's array (as opposed to exposing the Enum's own private one, which would open it to being modified).
– racraman
1 hour ago
FYI, theenum
facility in Java (based onEnum
class) is usually just called “enum” or “enum type” to avoid confusion with the interfacejava.util.Enumeration
.
– Basil Bourque
1 hour ago
add a comment |
2
You can certainly cache the length statically. Though I don't see where the array comes in. If you want to mimic a bit field, considerEnumSet
.
– shmosel
2 hours ago
2
stackoverflow.com/a/32354397/2970947
– Elliott Frisch
2 hours ago
1
Further to @ElliotFrisch's link, callingvalues()
does create a copy of the enum's array (as opposed to exposing the Enum's own private one, which would open it to being modified).
– racraman
1 hour ago
FYI, theenum
facility in Java (based onEnum
class) is usually just called “enum” or “enum type” to avoid confusion with the interfacejava.util.Enumeration
.
– Basil Bourque
1 hour ago
2
2
You can certainly cache the length statically. Though I don't see where the array comes in. If you want to mimic a bit field, consider
EnumSet
.– shmosel
2 hours ago
You can certainly cache the length statically. Though I don't see where the array comes in. If you want to mimic a bit field, consider
EnumSet
.– shmosel
2 hours ago
2
2
stackoverflow.com/a/32354397/2970947
– Elliott Frisch
2 hours ago
stackoverflow.com/a/32354397/2970947
– Elliott Frisch
2 hours ago
1
1
Further to @ElliotFrisch's link, calling
values()
does create a copy of the enum's array (as opposed to exposing the Enum's own private one, which would open it to being modified).– racraman
1 hour ago
Further to @ElliotFrisch's link, calling
values()
does create a copy of the enum's array (as opposed to exposing the Enum's own private one, which would open it to being modified).– racraman
1 hour ago
FYI, the
enum
facility in Java (based on Enum
class) is usually just called “enum” or “enum type” to avoid confusion with the interface java.util.Enumeration
.– Basil Bourque
1 hour ago
FYI, the
enum
facility in Java (based on Enum
class) is usually just called “enum” or “enum type” to avoid confusion with the interface java.util.Enumeration
.– Basil Bourque
1 hour ago
add a comment |
3 Answers
3
active
oldest
votes
EnumSet
"compose" these metrics into a single object
essentially bit flags
Sounds like you need a bit-array, with a bit flipped for the presence/absence of each predefined enum value.
If so, no need to roll your own. Use EnumSet
or EnumMap
. These are special implementations of the Set
and Map
interfaces. These classes are extremely efficient because of their nature handling enums, taking very little memory and being very fast to execute.
Take for example the built-in DayOfWeek
enum. Defines seven objects, one for each day of the week per ISO 8601 calendar.
Set< DayOfWeek > weekend = EnumSet.of( DayOfWeek.SATURDAY , DayOfWeek.SUNDAY ) ;
Use the convenient methods of Set
such as contains
.
boolean isTodayWeekend = weekend.contains( LocalDate.now().getDayOfWeek() ) ;
If you loop the elements of the set, they are promised to be provided in the order in which they are defined within the enum (their “natural” order). So, logically, an EnumSet
should have been marked as a SortedSet
, but mysteriously was not so marked. Nevertheless, you know the order. For example, looping EnumSet.allOf( DayOfWeek.class )
renders DayOfWeek.MONDAY
first and DayOfWeek.SUNDAY
last (per the ISO 8601 standard).
When Are Enum Values Defined?
The elements of an enum
are defined at compile-time, and cannot be modified at runtime. Each named variable is populated with an instance when the class is loaded. See Section 8.9, Enum Types of Java Language Specification.
If you define an enum Pet
with DOG
, CAT
, and BIRD
, then you know you will have exactly three instances at all times during runtime.
You can count the number of elements defined in an enum in at least two ways:
- Calling the
values
method generated by the compiler for anyenum
, where you can ask the size of the resulting array, as you show in your Question.int countDows = DayOfWeek.values().length() ;
- Calling
Set::size
after creating anEnumSet
of all enum instances.int countDows = EnumSet.allOf( DayOfWeek.class ).size() ;
1
@DarinBeaudreau You will findEnumSet
(andEnumMap
) quite useful. Notice the methods to copy, invert (complementOf
), empty (noneOf
&removeAll
), and quickly define a subset of a large number of elements (range
).
– Basil Bourque
1 hour ago
add a comment |
private static final int METRIC_COUNT = Metrics.values().length;
Can I do this?
Yes, you can. This is exactly the right way to cache the length and ensure it's only computed once.
I know the static variable will be determined at runtime (unless the value assigned to it were a literal value), so would the program be able to request the members of the Metrics enum at this point in execution?
Yep. As defined, METRIC_COUNT
is also computed at runtime.
add a comment |
I don't know if you need to do this in this case, but I think I understand the more general problem of not being able to compute a constant early in your execution. I've had this problem. The solution I've used is something like this...compute METRICS_COUNT later on, when you're ready to use it and are sure that the Metrics object is defined, but still only once:
private static int METRIC_COUNT = -1;
public static int getMetricsCount() {
if (METRIC_COUNT < 0)
METRIC_COUNT = Metrics.values().length;
return METRIC_COUNT;
}
Note that I'm not addressing the Enum problem specifically, as others are in the comments. I'm just proposing a way of putting off setting of a global value until just when it is needed.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54877109%2fwhen-are-enum-values-defined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
EnumSet
"compose" these metrics into a single object
essentially bit flags
Sounds like you need a bit-array, with a bit flipped for the presence/absence of each predefined enum value.
If so, no need to roll your own. Use EnumSet
or EnumMap
. These are special implementations of the Set
and Map
interfaces. These classes are extremely efficient because of their nature handling enums, taking very little memory and being very fast to execute.
Take for example the built-in DayOfWeek
enum. Defines seven objects, one for each day of the week per ISO 8601 calendar.
Set< DayOfWeek > weekend = EnumSet.of( DayOfWeek.SATURDAY , DayOfWeek.SUNDAY ) ;
Use the convenient methods of Set
such as contains
.
boolean isTodayWeekend = weekend.contains( LocalDate.now().getDayOfWeek() ) ;
If you loop the elements of the set, they are promised to be provided in the order in which they are defined within the enum (their “natural” order). So, logically, an EnumSet
should have been marked as a SortedSet
, but mysteriously was not so marked. Nevertheless, you know the order. For example, looping EnumSet.allOf( DayOfWeek.class )
renders DayOfWeek.MONDAY
first and DayOfWeek.SUNDAY
last (per the ISO 8601 standard).
When Are Enum Values Defined?
The elements of an enum
are defined at compile-time, and cannot be modified at runtime. Each named variable is populated with an instance when the class is loaded. See Section 8.9, Enum Types of Java Language Specification.
If you define an enum Pet
with DOG
, CAT
, and BIRD
, then you know you will have exactly three instances at all times during runtime.
You can count the number of elements defined in an enum in at least two ways:
- Calling the
values
method generated by the compiler for anyenum
, where you can ask the size of the resulting array, as you show in your Question.int countDows = DayOfWeek.values().length() ;
- Calling
Set::size
after creating anEnumSet
of all enum instances.int countDows = EnumSet.allOf( DayOfWeek.class ).size() ;
1
@DarinBeaudreau You will findEnumSet
(andEnumMap
) quite useful. Notice the methods to copy, invert (complementOf
), empty (noneOf
&removeAll
), and quickly define a subset of a large number of elements (range
).
– Basil Bourque
1 hour ago
add a comment |
EnumSet
"compose" these metrics into a single object
essentially bit flags
Sounds like you need a bit-array, with a bit flipped for the presence/absence of each predefined enum value.
If so, no need to roll your own. Use EnumSet
or EnumMap
. These are special implementations of the Set
and Map
interfaces. These classes are extremely efficient because of their nature handling enums, taking very little memory and being very fast to execute.
Take for example the built-in DayOfWeek
enum. Defines seven objects, one for each day of the week per ISO 8601 calendar.
Set< DayOfWeek > weekend = EnumSet.of( DayOfWeek.SATURDAY , DayOfWeek.SUNDAY ) ;
Use the convenient methods of Set
such as contains
.
boolean isTodayWeekend = weekend.contains( LocalDate.now().getDayOfWeek() ) ;
If you loop the elements of the set, they are promised to be provided in the order in which they are defined within the enum (their “natural” order). So, logically, an EnumSet
should have been marked as a SortedSet
, but mysteriously was not so marked. Nevertheless, you know the order. For example, looping EnumSet.allOf( DayOfWeek.class )
renders DayOfWeek.MONDAY
first and DayOfWeek.SUNDAY
last (per the ISO 8601 standard).
When Are Enum Values Defined?
The elements of an enum
are defined at compile-time, and cannot be modified at runtime. Each named variable is populated with an instance when the class is loaded. See Section 8.9, Enum Types of Java Language Specification.
If you define an enum Pet
with DOG
, CAT
, and BIRD
, then you know you will have exactly three instances at all times during runtime.
You can count the number of elements defined in an enum in at least two ways:
- Calling the
values
method generated by the compiler for anyenum
, where you can ask the size of the resulting array, as you show in your Question.int countDows = DayOfWeek.values().length() ;
- Calling
Set::size
after creating anEnumSet
of all enum instances.int countDows = EnumSet.allOf( DayOfWeek.class ).size() ;
1
@DarinBeaudreau You will findEnumSet
(andEnumMap
) quite useful. Notice the methods to copy, invert (complementOf
), empty (noneOf
&removeAll
), and quickly define a subset of a large number of elements (range
).
– Basil Bourque
1 hour ago
add a comment |
EnumSet
"compose" these metrics into a single object
essentially bit flags
Sounds like you need a bit-array, with a bit flipped for the presence/absence of each predefined enum value.
If so, no need to roll your own. Use EnumSet
or EnumMap
. These are special implementations of the Set
and Map
interfaces. These classes are extremely efficient because of their nature handling enums, taking very little memory and being very fast to execute.
Take for example the built-in DayOfWeek
enum. Defines seven objects, one for each day of the week per ISO 8601 calendar.
Set< DayOfWeek > weekend = EnumSet.of( DayOfWeek.SATURDAY , DayOfWeek.SUNDAY ) ;
Use the convenient methods of Set
such as contains
.
boolean isTodayWeekend = weekend.contains( LocalDate.now().getDayOfWeek() ) ;
If you loop the elements of the set, they are promised to be provided in the order in which they are defined within the enum (their “natural” order). So, logically, an EnumSet
should have been marked as a SortedSet
, but mysteriously was not so marked. Nevertheless, you know the order. For example, looping EnumSet.allOf( DayOfWeek.class )
renders DayOfWeek.MONDAY
first and DayOfWeek.SUNDAY
last (per the ISO 8601 standard).
When Are Enum Values Defined?
The elements of an enum
are defined at compile-time, and cannot be modified at runtime. Each named variable is populated with an instance when the class is loaded. See Section 8.9, Enum Types of Java Language Specification.
If you define an enum Pet
with DOG
, CAT
, and BIRD
, then you know you will have exactly three instances at all times during runtime.
You can count the number of elements defined in an enum in at least two ways:
- Calling the
values
method generated by the compiler for anyenum
, where you can ask the size of the resulting array, as you show in your Question.int countDows = DayOfWeek.values().length() ;
- Calling
Set::size
after creating anEnumSet
of all enum instances.int countDows = EnumSet.allOf( DayOfWeek.class ).size() ;
EnumSet
"compose" these metrics into a single object
essentially bit flags
Sounds like you need a bit-array, with a bit flipped for the presence/absence of each predefined enum value.
If so, no need to roll your own. Use EnumSet
or EnumMap
. These are special implementations of the Set
and Map
interfaces. These classes are extremely efficient because of their nature handling enums, taking very little memory and being very fast to execute.
Take for example the built-in DayOfWeek
enum. Defines seven objects, one for each day of the week per ISO 8601 calendar.
Set< DayOfWeek > weekend = EnumSet.of( DayOfWeek.SATURDAY , DayOfWeek.SUNDAY ) ;
Use the convenient methods of Set
such as contains
.
boolean isTodayWeekend = weekend.contains( LocalDate.now().getDayOfWeek() ) ;
If you loop the elements of the set, they are promised to be provided in the order in which they are defined within the enum (their “natural” order). So, logically, an EnumSet
should have been marked as a SortedSet
, but mysteriously was not so marked. Nevertheless, you know the order. For example, looping EnumSet.allOf( DayOfWeek.class )
renders DayOfWeek.MONDAY
first and DayOfWeek.SUNDAY
last (per the ISO 8601 standard).
When Are Enum Values Defined?
The elements of an enum
are defined at compile-time, and cannot be modified at runtime. Each named variable is populated with an instance when the class is loaded. See Section 8.9, Enum Types of Java Language Specification.
If you define an enum Pet
with DOG
, CAT
, and BIRD
, then you know you will have exactly three instances at all times during runtime.
You can count the number of elements defined in an enum in at least two ways:
- Calling the
values
method generated by the compiler for anyenum
, where you can ask the size of the resulting array, as you show in your Question.int countDows = DayOfWeek.values().length() ;
- Calling
Set::size
after creating anEnumSet
of all enum instances.int countDows = EnumSet.allOf( DayOfWeek.class ).size() ;
edited 1 hour ago
answered 1 hour ago


Basil BourqueBasil Bourque
112k28386545
112k28386545
1
@DarinBeaudreau You will findEnumSet
(andEnumMap
) quite useful. Notice the methods to copy, invert (complementOf
), empty (noneOf
&removeAll
), and quickly define a subset of a large number of elements (range
).
– Basil Bourque
1 hour ago
add a comment |
1
@DarinBeaudreau You will findEnumSet
(andEnumMap
) quite useful. Notice the methods to copy, invert (complementOf
), empty (noneOf
&removeAll
), and quickly define a subset of a large number of elements (range
).
– Basil Bourque
1 hour ago
1
1
@DarinBeaudreau You will find
EnumSet
(and EnumMap
) quite useful. Notice the methods to copy, invert (complementOf
), empty (noneOf
& removeAll
), and quickly define a subset of a large number of elements (range
).– Basil Bourque
1 hour ago
@DarinBeaudreau You will find
EnumSet
(and EnumMap
) quite useful. Notice the methods to copy, invert (complementOf
), empty (noneOf
& removeAll
), and quickly define a subset of a large number of elements (range
).– Basil Bourque
1 hour ago
add a comment |
private static final int METRIC_COUNT = Metrics.values().length;
Can I do this?
Yes, you can. This is exactly the right way to cache the length and ensure it's only computed once.
I know the static variable will be determined at runtime (unless the value assigned to it were a literal value), so would the program be able to request the members of the Metrics enum at this point in execution?
Yep. As defined, METRIC_COUNT
is also computed at runtime.
add a comment |
private static final int METRIC_COUNT = Metrics.values().length;
Can I do this?
Yes, you can. This is exactly the right way to cache the length and ensure it's only computed once.
I know the static variable will be determined at runtime (unless the value assigned to it were a literal value), so would the program be able to request the members of the Metrics enum at this point in execution?
Yep. As defined, METRIC_COUNT
is also computed at runtime.
add a comment |
private static final int METRIC_COUNT = Metrics.values().length;
Can I do this?
Yes, you can. This is exactly the right way to cache the length and ensure it's only computed once.
I know the static variable will be determined at runtime (unless the value assigned to it were a literal value), so would the program be able to request the members of the Metrics enum at this point in execution?
Yep. As defined, METRIC_COUNT
is also computed at runtime.
private static final int METRIC_COUNT = Metrics.values().length;
Can I do this?
Yes, you can. This is exactly the right way to cache the length and ensure it's only computed once.
I know the static variable will be determined at runtime (unless the value assigned to it were a literal value), so would the program be able to request the members of the Metrics enum at this point in execution?
Yep. As defined, METRIC_COUNT
is also computed at runtime.
answered 1 hour ago
John KugelmanJohn Kugelman
244k54406457
244k54406457
add a comment |
add a comment |
I don't know if you need to do this in this case, but I think I understand the more general problem of not being able to compute a constant early in your execution. I've had this problem. The solution I've used is something like this...compute METRICS_COUNT later on, when you're ready to use it and are sure that the Metrics object is defined, but still only once:
private static int METRIC_COUNT = -1;
public static int getMetricsCount() {
if (METRIC_COUNT < 0)
METRIC_COUNT = Metrics.values().length;
return METRIC_COUNT;
}
Note that I'm not addressing the Enum problem specifically, as others are in the comments. I'm just proposing a way of putting off setting of a global value until just when it is needed.
add a comment |
I don't know if you need to do this in this case, but I think I understand the more general problem of not being able to compute a constant early in your execution. I've had this problem. The solution I've used is something like this...compute METRICS_COUNT later on, when you're ready to use it and are sure that the Metrics object is defined, but still only once:
private static int METRIC_COUNT = -1;
public static int getMetricsCount() {
if (METRIC_COUNT < 0)
METRIC_COUNT = Metrics.values().length;
return METRIC_COUNT;
}
Note that I'm not addressing the Enum problem specifically, as others are in the comments. I'm just proposing a way of putting off setting of a global value until just when it is needed.
add a comment |
I don't know if you need to do this in this case, but I think I understand the more general problem of not being able to compute a constant early in your execution. I've had this problem. The solution I've used is something like this...compute METRICS_COUNT later on, when you're ready to use it and are sure that the Metrics object is defined, but still only once:
private static int METRIC_COUNT = -1;
public static int getMetricsCount() {
if (METRIC_COUNT < 0)
METRIC_COUNT = Metrics.values().length;
return METRIC_COUNT;
}
Note that I'm not addressing the Enum problem specifically, as others are in the comments. I'm just proposing a way of putting off setting of a global value until just when it is needed.
I don't know if you need to do this in this case, but I think I understand the more general problem of not being able to compute a constant early in your execution. I've had this problem. The solution I've used is something like this...compute METRICS_COUNT later on, when you're ready to use it and are sure that the Metrics object is defined, but still only once:
private static int METRIC_COUNT = -1;
public static int getMetricsCount() {
if (METRIC_COUNT < 0)
METRIC_COUNT = Metrics.values().length;
return METRIC_COUNT;
}
Note that I'm not addressing the Enum problem specifically, as others are in the comments. I'm just proposing a way of putting off setting of a global value until just when it is needed.
edited 1 hour ago
answered 1 hour ago
SteveSteve
771111
771111
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54877109%2fwhen-are-enum-values-defined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3 VUzMpogf0E,Jd54SMrM
2
You can certainly cache the length statically. Though I don't see where the array comes in. If you want to mimic a bit field, consider
EnumSet
.– shmosel
2 hours ago
2
stackoverflow.com/a/32354397/2970947
– Elliott Frisch
2 hours ago
1
Further to @ElliotFrisch's link, calling
values()
does create a copy of the enum's array (as opposed to exposing the Enum's own private one, which would open it to being modified).– racraman
1 hour ago
FYI, the
enum
facility in Java (based onEnum
class) is usually just called “enum” or “enum type” to avoid confusion with the interfacejava.util.Enumeration
.– Basil Bourque
1 hour ago