Replace character with another only if repeated and not part of a word
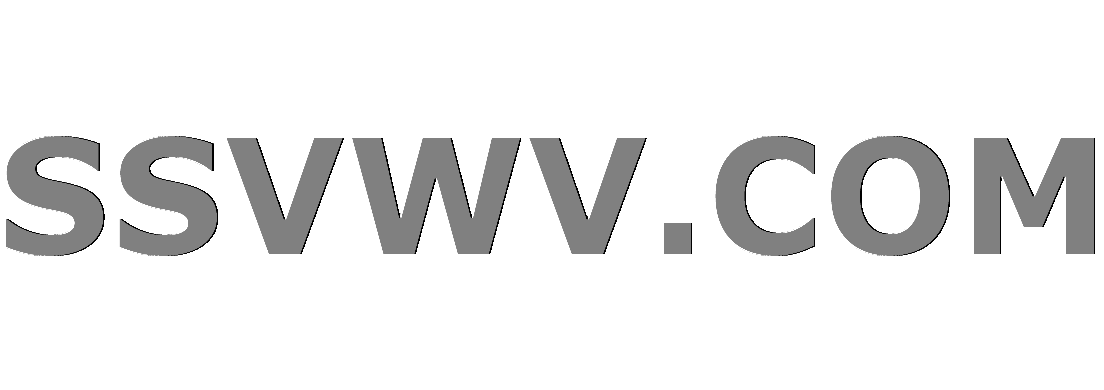
Multi tool use
In Python3, the following code works to replace a string (two or more) of *
's with x
's.
import re
re.sub(r'*(?=*)|(?<=*)*', 'x', 'Replace this *** but not this *')
# 'Replace this xxx but not this *'
But what if I also want to exempt a string of *
's that are part of a "word" like the following? (I.e. the string is attached to one or more [a-zA-Z]
characters.)
text = "Don't replace foo*** or **bar, either."
# unmodified text expected
How do I do this? I can probably match the exempted cases as well and use a replacement function to deal with them, but is there a better way?
python regex python-3.x
add a comment |
In Python3, the following code works to replace a string (two or more) of *
's with x
's.
import re
re.sub(r'*(?=*)|(?<=*)*', 'x', 'Replace this *** but not this *')
# 'Replace this xxx but not this *'
But what if I also want to exempt a string of *
's that are part of a "word" like the following? (I.e. the string is attached to one or more [a-zA-Z]
characters.)
text = "Don't replace foo*** or **bar, either."
# unmodified text expected
How do I do this? I can probably match the exempted cases as well and use a replacement function to deal with them, but is there a better way?
python regex python-3.x
add a comment |
In Python3, the following code works to replace a string (two or more) of *
's with x
's.
import re
re.sub(r'*(?=*)|(?<=*)*', 'x', 'Replace this *** but not this *')
# 'Replace this xxx but not this *'
But what if I also want to exempt a string of *
's that are part of a "word" like the following? (I.e. the string is attached to one or more [a-zA-Z]
characters.)
text = "Don't replace foo*** or **bar, either."
# unmodified text expected
How do I do this? I can probably match the exempted cases as well and use a replacement function to deal with them, but is there a better way?
python regex python-3.x
In Python3, the following code works to replace a string (two or more) of *
's with x
's.
import re
re.sub(r'*(?=*)|(?<=*)*', 'x', 'Replace this *** but not this *')
# 'Replace this xxx but not this *'
But what if I also want to exempt a string of *
's that are part of a "word" like the following? (I.e. the string is attached to one or more [a-zA-Z]
characters.)
text = "Don't replace foo*** or **bar, either."
# unmodified text expected
How do I do this? I can probably match the exempted cases as well and use a replacement function to deal with them, but is there a better way?
python regex python-3.x
python regex python-3.x
edited 3 hours ago


0m3r
8,02292554
8,02292554
asked 3 hours ago
bongbangbongbang
607720
607720
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
regex = r"s*{2,}[sn]"
This matches 2 or more *
chars, surrounded by whitespace (or ended with a newline)
Call it maybe like this?
regex = r"s*{2,}[sn]"
def replacer(match):
return 'x' * len(match.group())
re.sub(regex, replacer, your_string_here)
You gave me a good idea for the use of the replacer function, but your pattern, by including the spaces in the match, would seem to turnthis **** string
intothis******string
, which is not I want. It would also not replace**** this string
, which I do want to replace. That said, I now have a solution that works thanks to you. Thank you.
– bongbang
3 hours ago
add a comment |
This answer is inspired Danielle M.'s. This pattern below seems to give me what I want. The rest is the same as hers.
regex = r'(?<![a-zA-Z])*{2,}(?![a-zA-Z])'
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55389122%2freplace-character-with-another-only-if-repeated-and-not-part-of-a-word%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
regex = r"s*{2,}[sn]"
This matches 2 or more *
chars, surrounded by whitespace (or ended with a newline)
Call it maybe like this?
regex = r"s*{2,}[sn]"
def replacer(match):
return 'x' * len(match.group())
re.sub(regex, replacer, your_string_here)
You gave me a good idea for the use of the replacer function, but your pattern, by including the spaces in the match, would seem to turnthis **** string
intothis******string
, which is not I want. It would also not replace**** this string
, which I do want to replace. That said, I now have a solution that works thanks to you. Thank you.
– bongbang
3 hours ago
add a comment |
regex = r"s*{2,}[sn]"
This matches 2 or more *
chars, surrounded by whitespace (or ended with a newline)
Call it maybe like this?
regex = r"s*{2,}[sn]"
def replacer(match):
return 'x' * len(match.group())
re.sub(regex, replacer, your_string_here)
You gave me a good idea for the use of the replacer function, but your pattern, by including the spaces in the match, would seem to turnthis **** string
intothis******string
, which is not I want. It would also not replace**** this string
, which I do want to replace. That said, I now have a solution that works thanks to you. Thank you.
– bongbang
3 hours ago
add a comment |
regex = r"s*{2,}[sn]"
This matches 2 or more *
chars, surrounded by whitespace (or ended with a newline)
Call it maybe like this?
regex = r"s*{2,}[sn]"
def replacer(match):
return 'x' * len(match.group())
re.sub(regex, replacer, your_string_here)
regex = r"s*{2,}[sn]"
This matches 2 or more *
chars, surrounded by whitespace (or ended with a newline)
Call it maybe like this?
regex = r"s*{2,}[sn]"
def replacer(match):
return 'x' * len(match.group())
re.sub(regex, replacer, your_string_here)
answered 3 hours ago


Danielle M.Danielle M.
2,2051623
2,2051623
You gave me a good idea for the use of the replacer function, but your pattern, by including the spaces in the match, would seem to turnthis **** string
intothis******string
, which is not I want. It would also not replace**** this string
, which I do want to replace. That said, I now have a solution that works thanks to you. Thank you.
– bongbang
3 hours ago
add a comment |
You gave me a good idea for the use of the replacer function, but your pattern, by including the spaces in the match, would seem to turnthis **** string
intothis******string
, which is not I want. It would also not replace**** this string
, which I do want to replace. That said, I now have a solution that works thanks to you. Thank you.
– bongbang
3 hours ago
You gave me a good idea for the use of the replacer function, but your pattern, by including the spaces in the match, would seem to turn
this **** string
into this******string
, which is not I want. It would also not replace **** this string
, which I do want to replace. That said, I now have a solution that works thanks to you. Thank you.– bongbang
3 hours ago
You gave me a good idea for the use of the replacer function, but your pattern, by including the spaces in the match, would seem to turn
this **** string
into this******string
, which is not I want. It would also not replace **** this string
, which I do want to replace. That said, I now have a solution that works thanks to you. Thank you.– bongbang
3 hours ago
add a comment |
This answer is inspired Danielle M.'s. This pattern below seems to give me what I want. The rest is the same as hers.
regex = r'(?<![a-zA-Z])*{2,}(?![a-zA-Z])'
add a comment |
This answer is inspired Danielle M.'s. This pattern below seems to give me what I want. The rest is the same as hers.
regex = r'(?<![a-zA-Z])*{2,}(?![a-zA-Z])'
add a comment |
This answer is inspired Danielle M.'s. This pattern below seems to give me what I want. The rest is the same as hers.
regex = r'(?<![a-zA-Z])*{2,}(?![a-zA-Z])'
This answer is inspired Danielle M.'s. This pattern below seems to give me what I want. The rest is the same as hers.
regex = r'(?<![a-zA-Z])*{2,}(?![a-zA-Z])'
answered 3 hours ago
bongbangbongbang
607720
607720
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55389122%2freplace-character-with-another-only-if-repeated-and-not-part-of-a-word%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wwMZ,BKy5pdVnP3ZCcPD2eNGpj,DjKMvMGb7ZQzWIYCtc1VzVAJxi